
✒️ABAP La performance en ABAP
ABAP La performance en ABAP
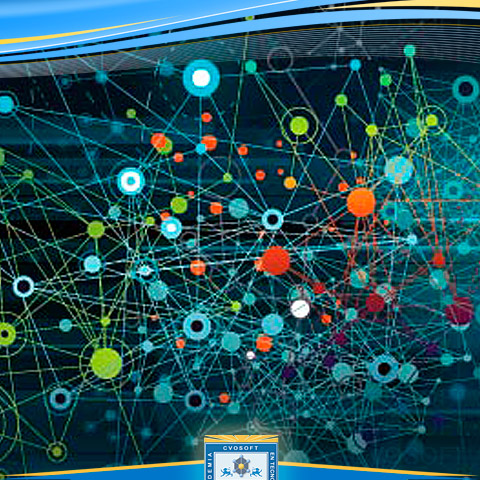
Performance in ABAP
Performance refers to the analysis of the execution and efficiency of ABAP programs. In this analysis, there are three fundamental aspects:
- The processing time of the program logic.
- The processing time of accesses to database tables.
- The processing time of the ABAP system.
Of these three times, the most resource-consuming one is the processing time of accesses to database tables, followed by the processing of logic, and finally the processing of the ABAP system.
For this analysis, we have the transaction SE30, which evaluates how the execution and processing time of the program are distributed.
To do this, upon entry, the name of the program is indicated, and the Evaluate button is pressed. This will show us how the percentage distribution of processing times for ABAP logic, the database, and the system.
The ideal situation would be for the highest percentage of processing to be the ABAP logic and the lowest to be the database table. To achieve this, it is necessary to adhere to good programming practices.
Good and Bad Practices in Database Access
- Avoid SELECT*: The SELECT* statement retrieves ALL data from the specified or indicated table. Instead of fetching all, it is suggested to specify which data needs to be retrieved, like so:
Wrong:
SELECT * FROM zuser_table INTO TABLE it_users.
Right:
SELECT user_id, username, email
FROM zuser_table
INTO TABLE it_users.
- Avoid SELECT ENDSELECT: The SELECT ENDSELECT statement generates a loop to process records retrieved from another database table. However, it's more efficient to use SELECT INTO TABLE.
Wrong:
SELECT * FROM zuser_table INTO TABLE it_users.
ENDSELECT.
Right:
SELECT user_id, username, email
FROM zuser_table
INTO TABLE it_users.
- Avoid SELECT without WHERE: When using the SELECT statement without a condition, ALL records from the database table are processed or fetched. It is ideal to use WHERE with as many specifications as possible to reduce processing to what is necessary. It is also suggested to avoid negative conditions or the use of NE.
Wrong:
SELECT * FROM zuser_table INTO TABLE it_users.
Right:
SELECT user_id, username, email
FROM zuser_table
WHERE is_active = 'X'
INTO TABLE it_users.
- Avoid SELECT within a LOOP: Executing one or more SELECT statements within a LOOP ENDLOOP will result in selecting each record while looping through the table. It's possible to use a SELECT SINGLE with specific conditions to select the first applicable case. Or retrieve all necessary records in internal tables first, then within the LOOP, access them using the READ TABLE statement. Additionally, retrieving all records from database table executes FOR ALL ENTRIES within the SELECT to retrieve records from the database into memory.
Wrong:
LOOP AT it_orders INTO wa_order.
SELECT * FROM zuser_table INTO wa_user WHERE user_id = wa_order-user_id.
" Process user data...
ENDLOOP.
Right:
SELECT * FROM zuser_table INTO TABLE @it_users WHERE user_id IN @it_orders-user_id.
LOOP AT it_orders INTO wa_order.
" Access user data from it_users table...
ENDLOOP.
- Avoid using INSERT, UPDATE, MODIFY, and DELETE statements within a LOOP: Doing this inside the LOOP will generate a longer table and require more resources. Instead, it is recommended to use these statements at the end or outside the LOOP.
Wrong:
LOOP AT it_items INTO wa_item.
INSERT INTO zitem_table VALUES wa_item.
ENDLOOP.
Right:
INSERT zitem_table FROM TABLE it_items.
- SELECT vs JOIN: When data from multiple database tables is required, using SELECT after SELECT is not recommended. Instead, use the JOIN statement as necessary. For example:
Wrong:
SELECT carrid, connid FROM spfli INTO TABLE @it_spfli.
SELECT dldate, planetype FROM sflight INTO TABLE @it_sflight.
Right:
SELECT tl~carrid, tl~connid, t2~dldate, t2~planetype, tl~countryfl
FROM spfli AS tl
INNER JOIN sflight AS t2
ON tl~carrid = t2~carrid AND tl~connid = t2~connid
INTO TABLE @it_combined_data.
Good and Bad Practices in ABAP Logic Processing
- READ TABLE BINARY SEARCH: When searching for a record in a table using the READ TABLE statement, the search is done sequentially. However, binary search partitions the table, searching in halves, offering better performance. To implement it, the search field in the internal table must be sorted ascendingly or descendingly, and add the BINARY SEARCH clause at the end of the READ TABLE:
Bad Practice:
READ TABLE it_suppliers INTO wa_suppliers WITH KEY name = 'Ariel'.
Good Practice:
SORT it_suppliers BY name ASCENDING.
READ TABLE it_suppliers INTO wa_suppliers WITH KEY name = 'Ariel' BINARY SEARCH.
- Avoid LOOP ENDLOOP within another LOOP ENDLOOP: Performing LOOPS within another LOOP generates an exponential search for records. It's suggested to use the WHERE conditional and binary search to optimize processing, like so:
Bad Practice:
LOOP AT it_suppliers INTO wa_suppliers.
LOOP AT it_users INTO wa_users.
" Processing logic
ENDLOOP.
ENDLOOP.
Good Practice:
SORT it_users BY name ASCENDING.
LOOP AT it_suppliers INTO wa_suppliers WHERE dni GT '50000000'.
READ TABLE it_users INTO wa_user WITH KEY dni = wa_suppliers-dni BINARY SEARCH.
ENDLOOP.
- LOOP CHECK vs LOOP WHERE: While CHECK and IF-ENDIF statements can be used as filters within a LOOP, they are not recommended as they read all records. It's optimal to implement WHERE with specific conditions.
Bad Practice:
LOOP AT it_customers INTO wa_customer.
CHECK wa_customer-status = 'Active'.
" Processing logic for active customers
ENDLOOP.
Good Practice:
LOOP AT it_customers INTO wa_customer WHERE status = 'Active'.
" Processing logic for active customers
ENDLOOP.
- Don't forget WHEN OTHERS in CASE statement: In the CASE-ENDCASE conditional, it's crucial to specify the case where none of the indicated cases are found, as otherwise, the system will throw an error or lead to unexpected situations. Implement the alternative WHEN OTHERS.
Bad Practice:
CASE lv_condition.
WHEN 'A'.
" Case A logic
WHEN 'B'.
" Case B logic
ENDCASE.
Good Practice:
CASE lv_condition.
WHEN 'A'.
" Case A logic
WHEN 'B'.
" Case B logic
WHEN OTHERS.
" Handling unexpected conditions
ENDCASE.
- APPEND from one internal table to another internal table: When adding records from one internal table to another of the same type, the worst option is to take the content of one table in a LOOP, transferring the information record by record to the other table. Instead, it's more efficient to use the APPEND LINES OF statement in a single line to transfer the content from one table to another, like so:
Bad Practice:
LOOP AT itab1 INTO wa_itab1.
APPEND wa_itab1 TO itab2.
ENDLOOP.
Good Practice:
APPEND LINES OF itab1 TO itab2.
- INSERT from one internal table to another internal table: When it's necessary to insert records from one internal table to another of the same type, the worst option is to insert record by record from one table to another. Instead, it's optimal to insert the content at a specific position from one table to another using the INSERT LINES OF statement, like so:
Bad Practice:
LOOP AT itab1 INTO wa_itab1.
INSERT wa_itab1 INTO TABLE itab2.
ENDLOOP.
Good Practice:
INSERT LINES OF itab1 INTO TABLE itab2 INDEX lv_index.
- Removing duplicate records from an internal table: When it's necessary to check and eliminate duplicate records from a table, primarily, the fields to be examined must be sorted. The worst option would be to check record by record if the first record looks like the next one and if so, delete it. However, it's possible to use the DELETE ADJACENT DUPLICATES statement along with COMPARING to compare the specified fields in this process.
Bad Practice:
DELETE ADJACENT DUPLICATES FROM itab.
Good Practice:
DELETE ADJACENT DUPLICATES FROM itab COMPARING field1 field2.
- Copying internal tables: When it's necessary to copy records from one internal table to another of the same type, the worst option is to clear the content of one table to pass the information from the other there. However, it's optimal to assign table1[] = table2[], overriding the content of one table with another.
Bad Practice:
CLEAR itab2.
LOOP AT itab1 INTO wa_itab1.
APPEND wa_itab1 TO itab2.
ENDLOOP.
Good Practice:
itab2[] = itab1[].
- Comparison of internal tables: When it's necessary to compare two internal tables to determine if their content is the same, the worst way is to do it manually. However, there's an optimal way to carry out this task using an IF-ENDIF within which the tables will be assigned, and in a single line, it will be determined if there's a duplicate, like so:
Bad Practice:
LOOP AT itab1 INTO wa_itab1.
READ TABLE itab2 INTO wa_itab2 WITH KEY field1 = wa_itab1-field1.
IF sy-subrc <> 0.
" Records not matching
ENDIF.
ENDLOOP.
Good Practice:
IF itab1[] = itab2[].
" Processing logic for equal tables
ENDIF.
 
 
 
Sobre el autor
Publicación académica de Jaime Eduardo Gomez Arango, en su ámbito de estudios para la Carrera Consultor ABAP.
Jaime Eduardo Gomez Arango
Profesión: Ingeniero de Sistemas y Computación - España - Legajo: SW34C
✒️Autor de: 99 Publicaciones Académicas
🎓Cursando Actualmente: Consultor ABAP Nivel Avanzado
🎓Egresado del módulo:
Disponibilidad Laboral: FullTime
Presentación:
Ingeniero de sistemas y computación con 8 años de experiencia el desarrollo frontend & backend (react/node) y en cloud (aws), actualmente desarrollando habilidades en sap btp, ui5, abap y fiori.
Certificación Académica de Jaime Gomez